-
This course will help you learn the core programming concepts and make you ready to write programs to solve complex problems.
- This course includes hands-on practice and will give you a solid knowledge of the Java language. After completing this course, you will be able to identify Java’s benefits, understand the code behind various websites and start programming in Java.
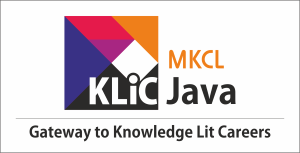
KLiC Java
Take your first step towards a career in software development with this course on Java Programming - one of the most in-demand programming languages.
Introduction
Who Should Join
- Aspiring Software Developers looking to pursue a career in software development, particularly in creating Java-based applications, web development, and database integration.
- Students in Computer Science or IT who need to strengthen their understanding of core programming concepts, object-oriented programming, and Java-specific technologies.
- Java Developers / Programmers already familiar with basic programming but seeking to deepen their knowledge of Java, including advanced topics like multithreading, collections, JDBC, and JSP.
- Web Developers interested in using Java for server-side development, JSP, servlets, and interacting with databases to create dynamic web applications.
- Full-Stack Developers who want to add Java back-end skills to their portfolio, integrating it with front-end technologies, databases, and web development tools.
- Software Engineers Preparing for Certifications like (Oracle Certified Professional) and need to cover topics like Java Collections, JDBC, and Servlets in depth.
- Beginner Programmers new to programming who want to start with Java, a versatile and widely used programming language for web and desktop applications.
What you'll learn ?
- Core programming concepts like Data types, Variables, Operators, Control Structures, Loops, Arrays, Functions, Recursions, Classes and Objects, Method Overloading, Constructors, Inheritance, Polymorphism etc.
- Along with the core concepts, you will also learn Java Collection Framework, Strings in Java, Multithreading, LAMBDA Expressions.
- In this course, you will learn about Basics of Databases & MySql, JDBC (Java Database Connectivity), Streams in Java, Java Applets, AWT Controls, Frame Projects, Java Swings
- This course focuses on creating Java projects and so includes introduction to Web Development, Using Java classes in JSP, Interacting with HTML Forms, Sessions and Cookies in JSP, JSP standard tag library (JSTL), Introduction to Servlets, Interaction between multiple pages, etc.
Certification
- KLiC courses are recognised by Yashwantrao Chavan Maharashtra Open University (YCMOU).
- MKCL provides certificate to the KLiC learner after his/her successful course completion.
- Yashwantrao Chavan Maharashtra Open University (YCMOU) provides mark sheet to successfully passed KLiC learners (Jurisdiction: Maharashtra).
Academic Approach
The academic approach of the courses focuses on the “work-centric” education i.e. begin with work (and not from a book!), derive knowledge from work and apply that knowledge to make the work more wholesome, useful and delightful. The ultimate objective is to empower the Learner to engage in socially useful and productive work. It aims at leading the learner to his/her rewarding career as an employee or entrepreneur as well as development of the community to which s/he belongs. Learning methodology:
- Step -1: Learners are given an overview of the course and its connection to life and work.
- Step -2: Learners are exposed to the specific tool(s) used in the course through the various real-life applications of the tool(s).
- Step -3: Learners are acquainted with the careers and the hierarchy of roles they can perform at workplaces after attaining increasing levels of mastery over the tool(s).
- Step -4: Learners are acquainted with the architecture of the tool or tool map so as to appreciate various parts of the tool, their functions, utility and inter-relations.
- Step -5: Learners are exposed to simple application development methodology by using the tool at the beginner’s level.
- Step -6: Learners perform the differential skills related to the use of the tool to improve the given ready-made industry-standard outputs.
- Step -7: Learners are engaged in appreciation of real-life case studies developed by the experts.
- Step -8: Learners are encouraged to proceed from appreciation to imitation of the experts.
- Step -9: After the imitation experience, they are required to improve the expert’s outputs so that they proceed from mere imitation to emulation.
- Step-10: Emulation is taken a level further from working with differential skills towards the visualization and creation of a complete output according to the requirements provided. (Long Assignments)
- Step-11: Understanding the requirements, communicating one’s own thoughts and presenting are important skills required in facing an interview for securing a work order/job. For instilling these skills, learners are presented with various subject-specific technical as well as HR-oriented questions and encouraged to answer them.
- Step-12: Finally, they develop the integral skills involving optimal methods and best practices to produce useful outputs right from scratch, publish them in their ePortfolio and thereby proceed from emulation to self-expression, from self-expression to self-confidence and from self-confidence to self-reliance and self-esteem!
Syllabus
- Why should you learn Java?
- Prerequisites for this course
- What are you going to learn?
- Introduction to programming languages
- Installing Java Development Kit (JDK)
- JDK, JRE and JVM
- Importance of Java in the context of Internet
- Features of Java
- Object Oriented Programming Paradigm (OOP)
- Relationship between classes and objects
- Features of OOPs - Encapsulation and Inheritance
- Features of OOPs - Abstraction and Polymorphism
- Data types in Java - Integers, floating-point, characters, Boolean
- Concept of variables, their declarations
- Literals - Integer, floating-point, character, Boolean, strings
- How to Write java code, compile and execute?
- Explanation of System class and printing method
- First Java Program using Notepad
- Explanation of important terms like main(), public, void, static
- Comments in Java
- Understanding command line arguments
- Sample program of command line arguments
- Using parseInt() with command line arguments
- Using parseFloat() with command line arguments
- Introduction to IDEs
- Components of Netbeans
- Creating a project in Netbeans
- Exporting & importing projects
- Intro to Eclipse IDE
- Intro to IntelliJ IDE
- Online IDEs
- First Java Program using NetBeans
- Second Java program in NetBeans
- Understanding concatenation
- Coding examples of concatenation
- Using Command Line arguments in Java program written in NetBeans
- Reading values from keyboard using Scanner class object
- Tricks to prevent the skipping of String input value
- Implicit type casting
- Explicit type casting
- Sample programs
- Formatting output using printf()
- More about formatting output
- Formatting dates
- Operators in Java
- Precedence and associativity of operators
- Examples of precedence and associativity
- Sample programs illustrating usage of arithmetic operators
- Sample programs illustrating usage of relational operators
- Sample programs illustrating usage of logical operators
- Sample programs illustrating usage of unary operators
- Sample programs illustrating usage of ternary operator
- Compound assignment operators with sample programs
- Programming Construct Sequence
- Sample programs of sequence
- if statement
- Sample programs of if
- if else if ladder
- Sample programs of if else if ladder
- Nested if
- Sample programs of nested if
- Overview of switch case construct
- Sample programs of switch case
- Difference between if and switch case
- Practice program - Switch Case - To find out salary of employee
- Basics of loops and while loop
- sample programs of while loop
- while loop pre-tested property
- Basics of do...while loop
- while loop post-tested property
- Basics of for loop
- Sample programs of for loop
- For loop pre-tested property
- More features of for loop
- Infinite loops
- Using break statement
- Using continue statement
- Practice program - Factorial
- Practice program - Reverse a number
- Practice program - Palindrome number
- Practice program - Armstrong number
- Programs - using loops
- Programs - using nested loops
- Practice program - Prime number
- Practice program - Biggest among n numbers
- Practice program - finding the square roots of a range of numbers
- Practice program - finding the power of numbers
- Practice program - generating Fibonacci series
- Practice program - printing ASCII set
- Practice program - Number guessing game
- Introduction to nested loops
- Second program of nested loops
- Third program of nested loops
- Fourth program of nested loops
- Fifth program of nested loops
- Practice program - Printing multiple tables
- Practice program - Asking the user whether to continue printing factorials
- Practice program - Asking the user whether to continue printing tables
- Explanation of Integer methods - compareto, toString, valueOf
- Programs of compareTo, toString, valueOf
- Explanation of Integer methods - doubleValue, floatValue, equals
- Programs of doubleValue, floatValue, equals
- Explanation of Integer methods - Signum, Min, Max, parseInt
- Programs of Signum,Min, Max, parseInt
- Explanation of Integer methods - intValue, sum
- Programs of intValue, sum
- Explanation of float methods - compareTo, toString
- Programs of compareTo, toString
- Explanation of valueOf, doublevalue, equals, parseFloat
- Programs of valueOf, doubleValue, equals, parseFloat
- The basic concept of arrays
- Declaring an array dynamically using two methods
- Traversing array elements
- Array name-reference to the array
- Using for each format of the for loop
- Merging two arrays
- Getting the absolute values of array elements
- Array of strings
- Linear Search Logic and algorithm
- Linear search program
- Logic of Binary search
- Algorithm of binary search
- Program of binary search
- Flow of Logic of Bubble sort
- Algorithm of bubble sort
- Bubble sort program
- Flow of logic of selection sort
- Algorithm of selection sort
- Selection sort program
- Using sort method of Array class
- Basics of double dimensional arrays
- First sample program of 2-D arrays
- Second sample program of 2-D arrays
- Third sample program of 2-D arrays
- 2-D arrays with different number of columns
- Matrix addition - logic and algo
- Program of matrix addition
- Logic of matrix multiplication
- Algorithm of matrix multiplication
- Program of matrix multiplication
- Basics of functions
- Components of a function
- Understanding actual & formal parameters - explanation
- Understanding actual & formal parameters - sample program
- Second sample program
- To return or not to return a value - sample programs
- Scope of variables in functions
- Facts about return statement
- Passing an array to a function - Explanation
- Passing an array to a function - example code
- Getting average age in an array
- Returning array reference
- Basics of recursion
- Basics of stacks
- Algo to push into a stack
- Algo to pop from a stack
- Factorial program through recursive function
- Practical coding of classes and objects
- Area of rectangle
- Area of circle
- Hospital billing
- Income tax calculation
- Creating an object within the class itself
- Array of references to objects - Company employees' example
- Array of references to objects - Exam result example
- The concept of null reference
- Assigning reference to another reference variable
- Automatic Garbage Collection
- Method Overloading (Compile-time polymorphism)
- Method overloading - Rainfall recording data
- Constructors in Java
- Default constructors
- Constructor overloading
- Constructor overloading - contd.
- Documentation comments
- this keyword
- Basics of getters and setters
- Using getters and setters for Office_rooms class
- Automatic creation of setter getter code
- Generating setters and getters for Pet_dogs class
- Generating setters and getters for Pet_dogs class
- static members of a class
- Counting number of objects - static members
- Basics of Packages
- Access specifiers (Visibility modes) in Java
- Understanding default and private members practically
- Same package classes in different programs
- same package classes in different programs - contd.
- Working in different packages
- usage of import keyword of Java
- usage of import keyword of Java - contd.
- Method signatures
- Creating abstract classes
- Abstract class Trainers
- The basics of interfaces
- Practical coding of interfaces
- Interface for areas and circumference
- An interface extending another interface
- Introduction to Inheritance
- Syntax of applying inheritance and Method overriding
- Writing the Java code for Inheritance
- Multi-Level inheritance
- Behaviour of constructors in inheritance
- Practical example of constructors without arguments in inheritance
- Practical example of constructors with arguments in inheritance
- Final classes
- Final methods
- Assigning reference of sub-class object into super class reference variable
- Abstract methods
- Basics of Polymorphism
- Practical implementation of Polymorphism
- Basics of exception handling
- Uncaught exceptions
- Catching exceptions using try .... catch blocks
- Printing Exception Class Reference Variable
- Multiple catch blocks
- calling a method from within try block
- calling a method from within try block - Execution
- Using super class Exception
- Handling NullPointerException
- Handling NumberFormatException
- throw: throwing an exception manually
- throws: handling checked exceptions
- Nested try block
- Introduction to data structures
- Basics of Collections and ArrayList traversal using Iterator interface
- Traversing ArrayList elements using for each loop
- Storing user-defined objects and retrieving them using Iterators interface
- Storing user-defined objects and retrieving them using foreach loop
- Storing and retrieving objects of nations' capitals
- Using toArray() method of ArrayList class
- Basics of linked lists
- Explanation of logic of traversal of nodes using algorithm
- Programs of LinkedList Collection
- Programs of stacks
- Basics of trees
- Adding numeric elements in a TreeSet
- Adding string elements in a tree TreeSet
- Removing an element from a TreeSet
- Searching an element from a TreeSet
- Basics of queues
- Explanation of insertion operation of a queue using algorithm
- Explanation of deletion operation of a queue using algorithm
- Programs of queues
- ListIterator interface - Traversing collection elements in both directions
- Using sort method of Collections class
- Sorting user-defined objects
- Hashset explanation and example
- Hashset with numeric values
- Applying size and contains methods of HashSet class
- Applying remove() method of HashSet class
- Applying clear() and isEmpty() methods of HashSet class
- LinkedHashset class
Evaluation Pattern
Evaluation Pattern of KLiC Courses consists of 4 Sections as per below table:
Section No. | Section Name | Total Marks | Minimum Passing Marks |
---|---|---|---|
1 | Learning Progression | 25 | 10 |
2 | Internal Assessment | 25 | 10 |
3 | Final Online Examination | 50 | 20 |
Total | 100 | 40 | |
4 | SUPWs (Socially Useful and Productive Work in form of Assignments) | 5 Assignments | 2 Assignments to be Completed & Uploaded |
YCMOU Mark Sheet
Printed Mark Sheet will be issued by YCMOU on successful completion of Section 1, Section 2 and Section 3 and will be delivered to the learner by MKCL.
YCMOU Mark Sheet will be available only for Maharashtra jurisdiction learners
MKCL's KLiC Certificate
The certificate will be provided to the learner who will satisfy the below criteria:
- Learners who have successfully completed above mentioned 3 Sections i.e. Section 1, Section 2 and Section 3
- Additionally, learner should have completed Section 4 (i.e. Section 4 will comprise of SUPWs i.e. Socially Useful and Productive Work in form of Assignments)
- Learner has to complete and upload minimum 2 out of 5 Assignments
KLiC Courses Fee Structure from 01 January, 2025
From 01 January 2025 onwards, the fees for all KLiC courses in ALCs of Mumbai Metropolitan Regional Development Authority (MMRDA), Pune Metropolitan Regional Development Authority (PMRDA) and Rest of Maharashtra will be applicable as shown in the table below:
KLiC Courses of 120 Hours:
Mode | Total Fee (Rupees) |
Single Installment (Rupees) |
Two Installments (Rupees) |
Single Installment | 6000/- | 6000/- | N/A |
Two Installments | 6200/- | 3100/- | 3100/- |
Total fee is including of Course fees, Examination fees and Certification fees
* Above mentioned fee is applicable for all Modes of KLiC Courses offered at Authorised Learning Center (ALC) and at Satellite Center
* Total fee is including of Course fees, Examination fees and Certification fees
* MKCL reserves the right to modify the Fees of Courses during the year without any prior notice and MKCL shall not be liable to anyone for any such modification/s